Bio
Hello, my name is Mobi Wan, and I'm a Prolific Java programmer with 4+ years of experience.
Badges
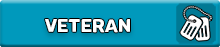
Activity Feed
Replied to thread : Reverse a String in python
Thank you so much man really appreciate your help and time.
Created a new thread : Reverse a String in python
I'm writing a Python software that writes out the entire song '99 bottles of beer' in reverse. The only thing I can't reverse are the numbers, which are integers rather than strings.
This is my entire script,
def reverse(str):
return str[::-1]
def plural(word, b):
if b != 1:
return word + 's'
else:
return word
def line(b, ending):
print b or reverse('No more'), plural(reverse('bottle'), b), reverse(ending)
for i in range(99, 0, -1):
line(i, "of beer on the wall")
line(i, "of beer"
print reverse("Take one down, pass it around")
line(i-1, "of beer on the wall \n")
I understand that my reverse function accepts a text as an argument after reading this article, but I don't know how to take in an integer or how to reverse the number later in the script.
Thank you in Advance for your help
Created a new thread : Fibonacci series
This function accepts the number of terms in the Fibonacci sequence in the child process, creates an array, and redirects the output to the parent via pipe. Parent must wait till the child develops the Fibonacci series. The received text always displays -1, although the transmitted text displays the number of entered numbers *4, which is acceptable.
#include<stdio.h>
#include<unistd.h>
#include<sys/types.h>
#include<string.h>
int* fibo(int n)
{
int* a=(int*)malloc(n*sizeof(int));
*(a+0)=0;
*(a+1)=1;
int i;
for(i=0;i<n-2;i++)
{
*(a+i+2)=*(a+i)+(*(a+i+1));
}
return a;
}
int main()
{
int* fib;
int fd[2];
pid_t childpid;
int n,nb;
int k=pipe(fd);
if(k==-1)
{
printf("Pipe failed");
return 0;
}
childpid=fork();
if(childpid == 0)
{
printf("Enter no. of fibonacci numbers");
scanf("%d",&n);
fib=fibo(n);
close(fd[0]);
nb=(fd[1],fib,n*sizeof(int));
printf("Sent string: %d \n",nb);
exit(0);
}
else
{
wait();
close(fd[1]);
nb= read(fd[0],fib,n*sizeof(int));
printf("Received string: %d ",nb);
}
return 0;
}
Created a new thread : A confusing situation in python
Okay so its abaout type casting in python. i was reading an article about this here and eventually grow a doubt.
Here's my predicament. I have a class structure similar to Django.
class Base:
...some stuff...
class Derived(Base):
...some more stuff...
Now, whenever I run a query in Django, I always receive objects of the Base type.
baseobj = get_object_or_404(Base, id = sid)
At runtime, I may find "Derived" objects with additional attributes. I can tell whether an item is base or derived (there is sufficient info in Base class object). But how can I get to those extra fields that are only available in the Derived class? I can't downcast "Base" to "Derived". How should I deal with it?
Created a new thread : Questions about Copy constructor Java
I have a question about Java copy building. Consider the following group:
I can say new(Integer(other.id)) in the copy constructor to receive a new integer object being supplied to the constructor, but I can't say new T(other.data) since the compiler will complain I can't instantiate the type T. How can I ensure that when the generic item is copied, it does not just pass a reference, causing the two objects to share the underlying data?
Also, the getLinks function does a new and creates a new object of the list, but is that going to deep copy and build a new object of the things included in the list or will it only have references to the current objects list items, resulting in two lists both pointing to the same data? See the comments / code below. Thank you in advance for your assistance.
class DigraphNode<T>
{
Integer id;
T data;
ArrayList<DigraphNode<T> > links;
public DigraphNode(Integer i)
{
id = i;
links = new ArrayList<DigraphNode<T> >();
}
public DigraphNode(Integer i, T d)
{
id = i; data = d;
links = new ArrayList<DigraphNode<T> >();
}
public DigraphNode(DigraphNode<T> other)
{
other.id = new Integer(other.id);
other.data = other.data; // line in question
this.links=other.getLinks(); // also will create a new list with references
// or will it deep copy the items contained in the list?
// see getLinks() method below
}
public void setData (T d ) { data = d; }
public void addLink (DigraphNode<T> n) { links.add(n); }
public void addLinks (ArrayList<DigraphNode<T> > ns) { links.addAll(ns); }
public ArrayList<DigraphNode<T> > getLinks()
{
return new ArrayList<DigraphNode<T> >(links);
}
public void printNode()
{
System.out.print("Id: " + id + " links: ");
for ( DigraphNode<T> i : links )
{
System.out.print(i.id + " ");
}
System.out.println();
}
}
Created a new thread : Use of *args and **kwargs
As a result, I'm having trouble grasping the notion of *args and **kwargs.
So far, I have discovered:
*args = list of arguments - as positional arguments **kwargs = dictionary - whose keys become distinct keyword arguments and the values become values of these arguments
I'm not sure what programming task this would assist with.
Maybe:
Is it possible to pass lists and dictionaries as function parameters while also acting as a wildcard?
Is there a simple example of how *args and **kwargs are used?
In addition, the tutorial (source) I found just utilised the "*" and a variable name.
Are *args and **kwargs only placeholders, or do you actually utilise *args and **kwargs in the code?
Created a new thread : How can I use a Bash script to see if a software exists?
How would I check the existence of a program in such a way that it either returns an error and exits or continues with the script?
I'm reading this article from scaler academy and It appears to be simple, yet it has confounded me. Can someone help me out?
Created a new thread : When deployed to Heroku, python setup.py egg info did not run successfully.
Hi everybody,
I joined this group to learn more about web development. I am familiar with Java, C, and HTML, and am actively learning CSS and Python. And I'm facing some issue! Can somebody help me?
After deploying and hosting my website, I upgraded my pip version. Then I installed the pypaystack package. When I try to push to Heroku, I get the error shown below. In the development stage, the project is running smoothly.
Collecting pypaystack==1.24
remote: Downloading pypaystack-1.24.tar.gz (5.4 kB)
remote: Preparing metadata (setup.py): started
remote: Preparing metadata (setup.py): finished with status 'error'
remote: error: subprocess-exited-with-error
remote:
remote: × python setup.py egg_info did not run successfully.
remote: │ exit code: 1
remote: ╰─> [12 lines of output]
remote: Traceback (most recent call last):
remote: File "<string>", line 2, in <module>
remote: File "<pip-setuptools-caller>", line 34, in <module>
remote: File "/tmp/pip-install-h6ay7jb3/pypaystack_2a3b97aa3d934da4b6c7a7d81a4a6ad2/setup.py", line 2, in <module>
remote: from pypaystack import version
remote: File "/tmp/pip-install-h6ay7jb3/pypaystack_2a3b97aa3d934da4b6c7a7d81a4a6ad2/pypaystack/__init__.py", line 3, in <module>
remote: from .customers import Customer
remote: File "/tmp/pip-install-h6ay7jb3/pypaystack_2a3b97aa3d934da4b6c7a7d81a4a6ad2/pypaystack/customers.py", line 1, in <module>
remote: from .baseapi import BaseAPI
remote: File "/tmp/pip-install-h6ay7jb3/pypaystack_2a3b97aa3d934da4b6c7a7d81a4a6ad2/pypaystack/baseapi.py", line 2, in <module>
remote: import requests
remote: ModuleNotFoundError: No module named 'requests'
remote: [end of output]
remote:
remote: note: This error originates from a subprocess, and is likely not a problem with pip.
remote: error: metadata-generation-failed
remote:
remote: × Encountered error while generating package metadata.
remote: ╰─> See above for output.
remote:
remote: note: This is an issue with the package mentioned above, not pip.
remote: hint: See above for details.
remote: ! Push rejected, failed to compile Python app.
What can be the issue? I even created a new virtual environment but the error remains.
I'm hoping that this community will assist me on my path.
Thanks!